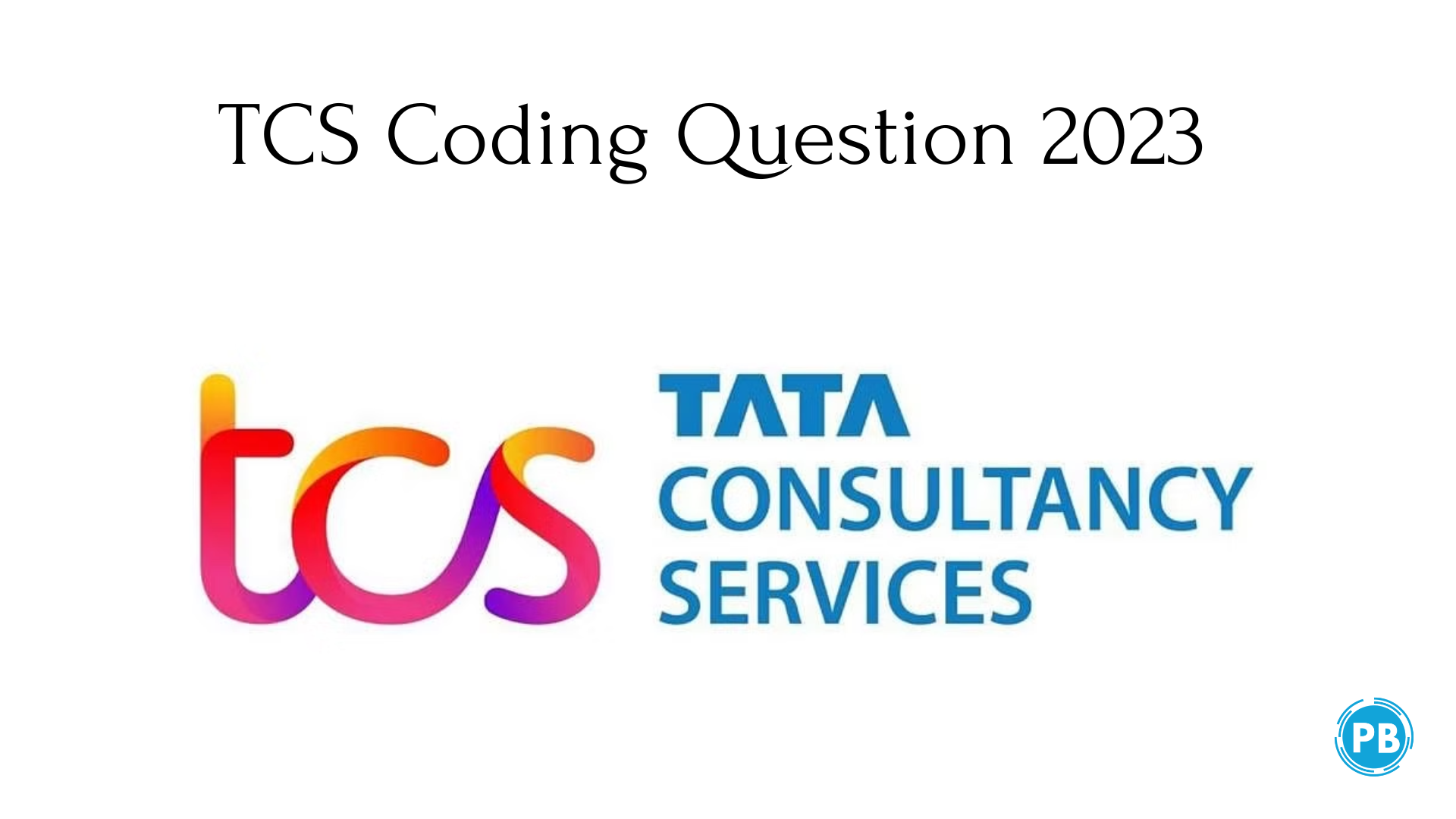
Problem Description -: Given an array Arr[ ] of N integers and a positive integer K. The task is to cyclically rotate the array clockwise by K.
Note: Keep the first of the array unaltered.
Example 1:
- 5 —Value of N
- {10, 20, 30, 40, 50} —Element of Arr[ ]
- 2 —–Value of K
Output :
40 50 10 20 30
Example 2:
- 4 —Value of N
- {10, 20, 30, 40} —Element of Arr[]
- 1 —–Value of K
Output :
40 10 20 30
C++
#include<bits/stdc++.h> using namespace std; vector rotate(int nums[], int n, int k) { if (k > n) k = k % n; vector ans(n); for (int i = 0; i < k; i++) { ans[i] = nums[n - k + i]; } int index = 0; for (int i = k; i < n; i++) { ans[i] = nums[index++]; } return ans; } int main() { int Array[] = { 1, 2, 3, 4, 5 }; int N = sizeof(Array) / sizeof(Array[0]); int K = 2; vector ans = rotate(Array, N, K); for (int i = 0; i < N; ++i) { cout << ans[i] << ' '; } }
Solution Explanation
This code is for rotating an array of numbers to the right by a given number of positions (K positions). Here's a simple explanation:
- We have an array called
Array
with some numbers and want to rotate it. N
is the number of elements in the array, andK
is the number of positions we want to rotate it to the right.- We create a function called
rotate
that takes three arguments: the arraynums
, its sizen
, and the number of positions to rotatek
.
Inside the rotate
function:
4. We check if k
is greater than n
, and if it is, we set k
to be the remainder of k
divided by n
. This is to handle cases where k
is larger than the array size.
- We create a vector called
ans
with the same size as the array to store the rotated elements. - We copy the last
k
elements from the original array to the beginning of theans
vector. - Then, we copy the remaining elements from the original array to the end of the
ans
vector.
Finally, in the main
function:
8. We call the rotate
function with our array Array
, its size N
, and the number of positions to rotate K
.
- We print the elements of the
ans
vector, which now contains the rotated array.
So, if you run this code with the given array [1, 2, 3, 4, 5]
and rotate it 2 positions to the right, it will print: 4 5 1 2 3
, which is the array after rotation.
JAVA
import java.util.*; public class Main { static int[] rotate(int nums[], int n, int k) { if (k > n) k = k % n; int[] ans = new int[n]; for (int i = 0; i < k; i++) { ans[i] = nums[n - k + i]; } int index = 0; for (int i = k; i < n; i++) { ans[i] = nums[index++]; } return ans; } public static void main(String[] args) { int Array[] = { 1, 2, 3, 4, 5 }; int N = 5; int K = 2; int[] ans = rotate(Array, N, K); for (int i = 0; i < N; ++i) { System.out.println(ans[i]); } } }
Solution Explanation
This Java code is for rotating an array of numbers to the right by a given number of positions (K positions). Here's a simple explanation:
- We have an array called
Array
with some numbers[1, 2, 3, 4, 5]
, and we want to rotate it. N
is set to5
, which is the number of elements in the array, andK
is set to2
, which is the number of positions we want to rotate the array to the right.
Inside the rotate
method: 3. We check if k
(the number of positions to rotate) is greater than n
(the size of the array). If it is, we set k
to be the remainder of k
divided by n
. This is to handle cases where k
is larger than the array size.
- We create an integer array called
ans
with the same size as the original array to store the rotated elements. - We copy the last
k
elements from the original array to the beginning of theans
array. - Then, we copy the remaining elements from the original array to the end of the
ans
array.
In the main
method: 7. We call the rotate
method with our array Array
, its size N
(which is 5), and the number of positions to rotate K
(which is 2).
- We store the result, the rotated array, in the
ans
array. - We then loop through the
ans
array and print each element usingSystem.out.println()
.
So, when you run this Java code with the given array [1, 2, 3, 4, 5]
and rotate it 2 positions to the right, it will print:
4
5
1
2
3
These are the elements of the array after rotation.
Do you dream of turning your thoughts and words into income ? join us now and become blogger now.
0 Comments
Leave a Comment